In this article, we will learn about securing applications with Spring Boot Security using OAuth2 with JWT. Here I explained in a detail with the help of a Real-time example.
Before moving forward I would like to briefly explain terms such as OAuth2 and JWT which will frequently use in a further section of this tutorial.
Note: Video Tutorial is available in the bottom of this article.
What is OAuth2?
It is an authorization framework that provides some standardized rules for authorization. It was first created in 2006 and the first version was OAuth.
The main goal of the OAuth2 framework is to provide a simple flow of authorization that can be implemented on the web application, mobile phones, desktop application, and even on the devices used in our living rooms.
OAuth2 specify the four roles on server side:
- Resource Owner.
- Resource Server.
- Authentication Server.
- Client.
Resource Owner: The person or any entity that can provide access to the protected resources.
Resource Server: It can be your application, which provides an access token to the end-user or client so that they can access the protected resource.
Note: Here protected resources refer to the API, web pages, etc which you developed or created, more technically we can say protected resources is HTTP Endpoints, the resources which are static.
Authorization Server: It is a centralized server that acts as an intermediate between the Resource owner and client. This server is only responsible for providing access to the client after successful authentication.
Note: It may be possible that the Resource Server and Authorization server can be the same. Single Authorization Server can provide access tokens to more than one resource server.
Client: It is the one who requested the Resource owner or Authorization Server to provide access to the protected resources such as Apis or HTTP Endpoints.
Here the client can be UI/UX also or when you search anything on the internet, then the URL which you enter on the browser will send some request to the Resource Server.
JWT Token
The full form of JWT is JSON Web Token. It is like an entry pass to the client which Authorization Server verify before providing access to protected resources such as API or HTTP Endpoints.
It is a proof which specifies that there is secure communication between client and server-side code or Resource Server.
Advantages of using OAuth2 with JWT
- It is more popular in case microservices architecture where the single authentication server can be used for multiple resources server.
- Since the format of the token is JSON so it can be easily understood and managed on the client-side.
Let us begin our practical OAuth2 implementation with JWT in our application. Now we are going to build a Spring Boot application where we enable all necessary Security features which we had to discuss till now.
You need to follow all mentioned steps, in order to build an application having Spring Boot Security using OAuth2 with JWT.
Step 1: Create a simple maven project from the Spring Initializr.
Note: If you learning Spring Boot Security then I am assuming that you know how to create and import a project from Spring Initalizr to IDE such as Eclipse. In case if you don’t know please check out the following article first Build the first Application with Spring Boot.
Step 2: Add the following dependencies on your pom.xml file. I explained below about each dependency in detail.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-jwt</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
Explanation of Dependencies used inside the pom.xml file
1. spring-boot-starter-web
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
The above dependency is used to write the HTTP Endpoints or to create an API in the RestController class.
2. spring-boot-starter-jdbc
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
It provides the communication of your server-side code with the database , in order to validate the user or client.
3. spring-boot-starter-security
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
With the help of security dependency, you can access all necessary packages to implements the spring security in your application.
4. spring-security-oauth2
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
</dependency>
It provides the OAuth2 framework using you can implement features such as Authorization Server and Resource Server on your application.
5. spring-security-jwt
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-jwt</artifactId>
</dependency>
It helps to generate the JSON Web Token, which further used by the client to access the protected resources.
6. com.h2database
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
</dependency>
It provides the in-memory database where you can store the client details for authentication and authorization.
7. spring-boot-starter-test
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
In order to test our spring boot application we required this dependency.
8. spring-security-test
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
Complete pom.xml file
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.2.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.pixelTrice</groupId>
<artifactId>firstSpringBootApplication</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>firstSpringBootApplication</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
<start-class>
com.pixelTrice.firstSpringBootApplication.FirstSpringBootApplication
</start-class>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.5.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-jwt</artifactId>
<version>1.1.1.RELEASE</version>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Step 3: Enable the Authorization Server and Resource Server
In the next step, we have to tag the SpringBootApplication or main class with @EnableAuthorizationServer and @EnableResourceServer annotation as shown below.
package com.pixelTrice.firstSpringBootApplication;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableAuthorizationServer;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer;
@SpringBootApplication
@EnableAuthorizationServer
@EnableResourceServer
public class FirstSpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(FirstSpringBootApplication.class, args);
}
}
@EnableAuthorizationServer: This annotation enables the Authorization Server in the application.
@EnableResourceServer: It makes the application Resource Server.
Note: In this case, our application will act as both Authorization as well as Resource Server.
Step 4: Create a Pojo Class
Here we will create a class or POJO class to store the users or client information in the database.
package com.pixelTrice.firstSpringBootApplication;
import java.util.ArrayList;
import java.util.Collection;
import org.springframework.security.core.GrantedAuthority;
public class UsersPojo {
private String username;
private String password;
private Collection<GrantedAuthority> listOfgrantedAuthorities = new ArrayList<>();
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public Collection<GrantedAuthority> getListOfgrantedAuthorities() {
return listOfgrantedAuthorities;
}
public void setListOfgrantedAuthorities(Collection<GrantedAuthority> listOfgrantedAuthorities) {
this.listOfgrantedAuthorities = listOfgrantedAuthorities;
}
}
If you noticed in the above code we declared three variables, the first two are very commonly used everywhere but the third one Collection<GrantedAuthority>. Let us understand this variable.
Collection<GrantedAuthority>: It represents the collection or list of Granted Authority.
GrantedAuthority: It is an interface in Spring Security which used to provides permission to perform some tasks to the user, for example, add the new user or delete the existing user, etc. I will explain with the code below it will be very easy to understand.
Step 5: Create a User Helper Class
We need to create one more class that extends the User class provided by spring security and it is present in org.springframework.security.core.userdetails.User.The purpose of creating a UsersHelper class is to authenticate the user.
package com.pixelTrice.firstSpringBootApplication;
import org.springframework.security.core.userdetails.User;
public class UsersHelper extends User{
private static final long serialVersionUID = 1L;
public UsersHelper(UsersPojo user) {
super(
user.getUsername(),
user.getPassword(),
user.getListOfgrantedAuthorities()
);
}
}
Step 6: Create a User DAO or Repository class
Let’s create a class to read the user’s details such as password, username, etc from the database and send it to the UsersHelper class for authentication. This class we also called DAO or Repository class. We need to tag this class with @Repository annotation.
package com.pixelTrice.firstSpringBootApplication;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.stereotype.Repository;
@Repository
public class UsersDBQuery {
@Autowired
private JdbcTemplate jdbcTemplate;
public UsersPojo getUserDetails(String username) {
Collection<GrantedAuthority> listOfgrantedAuthorities = new ArrayList<>();
String userSQLQuery = "SELECT * FROM USERS WHERE USERNAME=?";
List<UsersPojo> list = jdbcTemplate.query(userSQLQuery, new String[] { username },
(ResultSet rs, int rowNum) -> {
UsersPojo user = new UsersPojo();
user.setUsername(username);
user.setPassword(rs.getString("PASSWORD"));
return user;
});
if (list.size() > 0) {
GrantedAuthority grantedAuthority = new SimpleGrantedAuthority("ROLE_SYSTEMADMIN");
listOfgrantedAuthorities.add(grantedAuthority);
list.get(0).setListOfgrantedAuthorities(listOfgrantedAuthorities);
return list.get(0);
}
return null;
}
}
In the above class, we have written a query to fetch the user detail. For GrantedAuthority we have set the value ROLE_SYSTEMADMIN. It means the role of the user is System Admin and this user we able to perform all tasks inside the application which given to SYSTEM ADMIN.
Step 7: Create a UserDetail Service Class
We can also create UsersDetailService that extends the org.springframework.security.core.userdetails.UserDetailsService .The purpose of this class to call or invoke the DAO class or Repository class as shown below.
package com.pixelTrice.firstSpringBootApplication;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;
@Service
public class UsersService implements UserDetailsService {
@Autowired
UsersDBQuery usersDBQuery;
@Override
public UsersHelper loadUserByUsername(final String username) throws UsernameNotFoundException {
UsersPojo usersPojo = null;
try {
usersPojo = usersDBQuery.getUserDetails(username);
UsersHelper userDetail = new UsersHelper(usersPojo);
return userDetail;
} catch (Exception e) {
e.printStackTrace();
throw new UsernameNotFoundException("User " + username + " was not found in the database");
}
}
}
Step 8: Create a Spring Security Configuration class
Next, we need to create a configuration class that helps to enable Web Security. To make a configuration class, we need to tag the class with @Configuration annotation.
In this, we need to define two very important methods such as the encoder() and authenticationManagerBean(). And this class should extend the WebSecurityConfigurerAdapter class.
package com.pixelTrice.firstSpringBootApplication;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.method.configuration.EnableGlobalMethodSecurity;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.builders.WebSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
@Autowired
private UsersService usersService;
@Bean
public PasswordEncoder encoder() {
return new BCryptPasswordEncoder();
}
@Override
@Autowired
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(usersService).passwordEncoder(encoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests().anyRequest().authenticated().and().sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.NEVER);
}
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring();
}
@Override
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
}
public PasswordEncoder encoder() : This method return the object or bean of BCryptPasswordEncoder. Since it is returning the bean, so we tagged this method with @Bean annotation so that the spring container will manage this bean.
The purpose of this method is to convert the plain password into hash format during the registration of a new user or it converts to the hash format at the time of user authentication.
public AuthenticationManager authenticationManagerBean():
Step 9: Create OAuth2 Configuration Class.
Let’s create an OAuth2 configuration class to add the Private Key, Public Key for token signer key and verifier key, Client ID, Client Secret, and also define the JwtAccessTokenConverter and we will configure the ClientDetailsServiceConfigurer to validate the token.
package com.pixelTrice.firstSpringBootApplication;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.oauth2.config.annotation.configurers.ClientDetailsServiceConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configuration.AuthorizationServerConfigurerAdapter;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerEndpointsConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerSecurityConfigurer;
import org.springframework.security.oauth2.provider.token.store.JwtAccessTokenConverter;
import org.springframework.security.oauth2.provider.token.store.JwtTokenStore;
@Configuration
public class ConfigOAuth2 extends AuthorizationServerConfigurerAdapter{
private String clientId = "pixeltrice";
private String clientSecret = "pixeltrice-secret-key";
private String privateKey = "enter your private key after generating from openssl";
private String publicKey = "enter your public key";
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Autowired
PasswordEncoder passwordEncoder;
@Bean
public JwtAccessTokenConverter tokenEnhancer() {
JwtAccessTokenConverter converter = new JwtAccessTokenConverter();
converter.setSigningKey(privateKey);
converter.setVerifierKey(publicKey);
return converter;
}
@Bean
public JwtTokenStore tokenStore() {
return new JwtTokenStore(tokenEnhancer());
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager).tokenStore(tokenStore())
.accessTokenConverter(tokenEnhancer());
}
@Override
public void configure(AuthorizationServerSecurityConfigurer security) throws Exception {
security.tokenKeyAccess("permitAll()").checkTokenAccess("isAuthenticated()");
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory().withClient(clientId).secret(passwordEncoder.encode(clientSecret)).scopes("read", "write")
.authorizedGrantTypes("password", "refresh_token").accessTokenValiditySeconds(20000)
.refreshTokenValiditySeconds(20000);
}
}
Note: For generating the public key and private key I am using OpenSSL software if you don’t have then please download and install it. Once you completed the installation just open it, it will look similar to the command prompt as shown below.
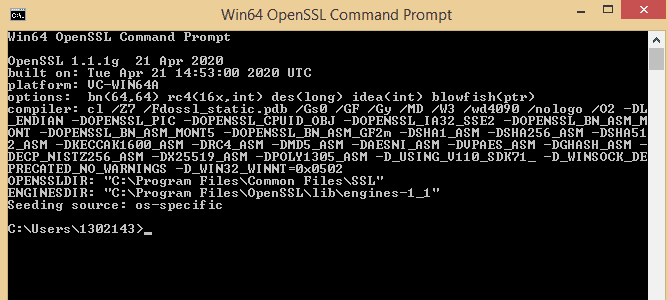
Let us create a public and private key with the following commands using OpenSSL Command prompt.
Command for generating the private key
openssl genrsa -out jwt.pem 2048
Type the above command as shown below.
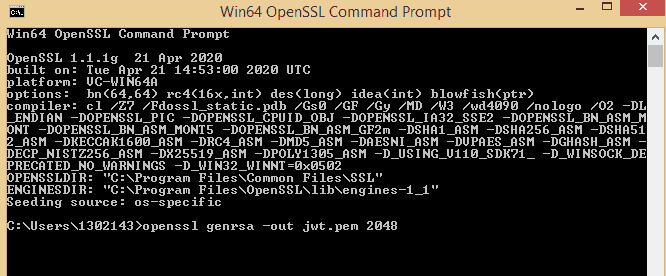
Once you press enter you will get the screen like below.
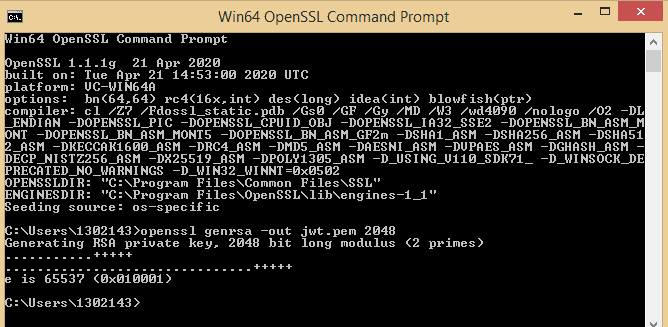
Enter the another command.
openssl rsa -in jwt.pem
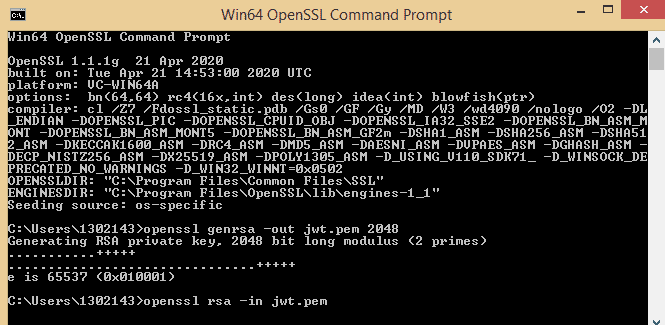
Press enter . You will get the private key as shown in the figure.
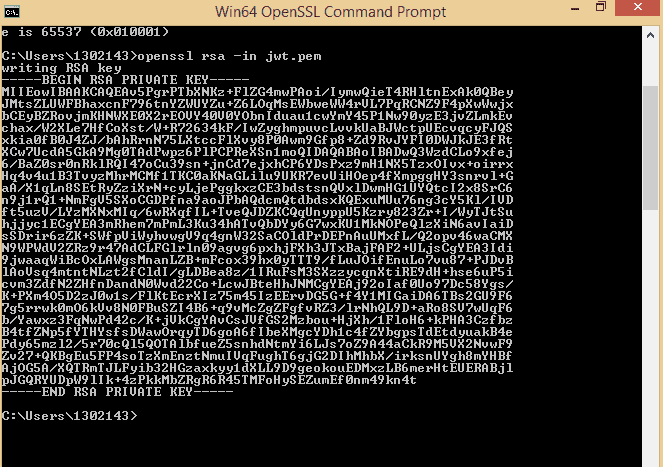
Copy the private key from —–BEGIN RSA PRIVATE KEY—– up to —–END RSA PRIVATE KEY—– and placed inside the double quote. I have attached the code below for ConfigOAuth2.java class
Command for creating the public key.
openssl rsa -in jwt.pem -pubout
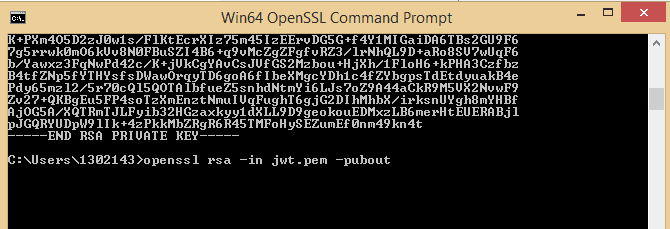
Once you hit enter after typing above command you will get the public key.
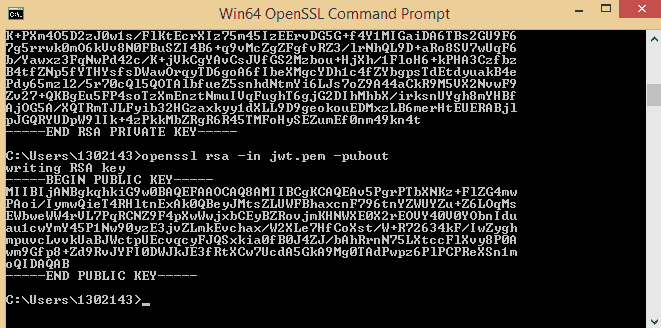
Please copy and paste inside the ConfigOAuth2.java class as shown below.
package com.pixelTrice.firstSpringBootApplication;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.oauth2.config.annotation.configurers.ClientDetailsServiceConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configuration.AuthorizationServerConfigurerAdapter;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerEndpointsConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerSecurityConfigurer;
import org.springframework.security.oauth2.provider.token.store.JwtAccessTokenConverter;
import org.springframework.security.oauth2.provider.token.store.JwtTokenStore;
@Configuration
public class ConfigOAuth2 extends AuthorizationServerConfigurerAdapter{
private String clientId = "pixeltrice";
private String clientSecret = "pixeltrice-secret-key";
private String privateKey = "-----BEGIN RSA PRIVATE KEY-----\r\n" + "MIIEowIBAAKCAQEAv5PgrPTbXNKz+FlZG4mwPAoi/IymwQieT4RHltnExAk0QBey\r\n" +
"-----END RSA PRIVATE KEY-----";
private String publicKey = "-----BEGIN PUBLIC KEY-----\r\n" +
"MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAv5PgrPTbXNKz+FlZG4mw\r\n" +
"-----END PUBLIC KEY-----";
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Autowired
PasswordEncoder passwordEncoder;
@Bean
public JwtAccessTokenConverter tokenEnhancer() {
JwtAccessTokenConverter converter = new JwtAccessTokenConverter();
converter.setSigningKey(privateKey);
converter.setVerifierKey(publicKey);
return converter;
}
@Bean
public JwtTokenStore tokenStore() {
return new JwtTokenStore(tokenEnhancer());
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager).tokenStore(tokenStore())
.accessTokenConverter(tokenEnhancer());
}
@Override
public void configure(AuthorizationServerSecurityConfigurer security) throws Exception {
security.tokenKeyAccess("permitAll()").checkTokenAccess("isAuthenticated()");
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory().withClient(clientId).secret(passwordEncoder.encode(clientSecret)).scopes("read", "write")
.authorizedGrantTypes("password", "refresh_token").accessTokenValiditySeconds(20000)
.refreshTokenValiditySeconds(20000);
}
}
Note: If you are using the Spring Boot version greater than 1.5 then please place the following line of code inside application.properties.
security.oauth2.resource.filter-order=3
Step 10: Create a Rest Controller class
In this class we will create a API or HTTP Endpoints as a Resources, so that once the client pass throgh authentication then they will able to access the API.
package com.pixelTrice.firstSpringBootApplication;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloWorldController {
@RequestMapping("/home")
public String index() {
return "Greetings from Spring Boot!";
}
}
All configuration part has done. Let us focus on creating a table inside the in-memory database(H2 database).
Step 1: Create a schema.sql file on the path src/main/resources and insert the following query to create a user table.
CREATE TABLE USERS (ID INT PRIMARY KEY, USERNAME VARCHAR(50), PASSWORD VARCHAR(70));
Step 2: Create a data.sql file on the path src/main/resources to insert some values inside the user’s table.
INSERT INTO USERS (ID, USERNAME,PASSWORD) VALUES (1, 'admin@pixeltrice.com','$2a$08$fL7u5xcvsZl78su29x1ti.dxI.9rYO8t0q5wk2ROJ.1cdR53bmaVG');
Note: Since we are using Bcrypt format to store the password. So, it should always be in the same format while inserting the value as you can see in the above insert query.
Final folder structure of code.
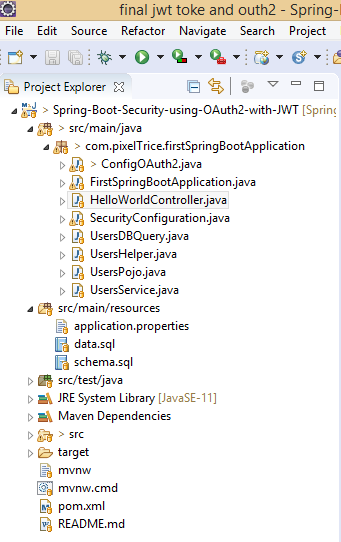
Create a jar file of the application
Step 1: Go inside the folder where the project is located. On my system, it is located on following path C:\Users\1302143\Desktop\firstSpringBootApplication
Step 2: Open the command prompt inside that folder, make sure you should on the following path in your command prompt as shown in the below figure.
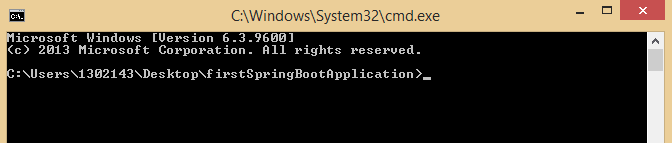
Step 3: Enter the command mvn clean install and press Enter.
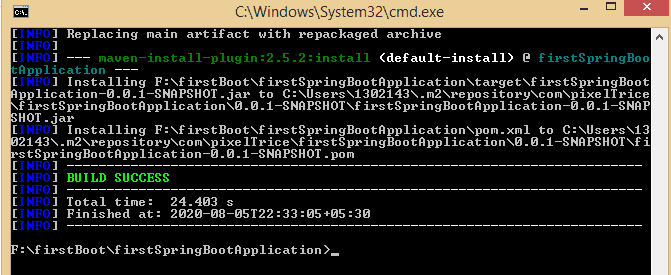
Jar file of the application has created Inside C:\Users\1302143\Desktop\firstSpringBootApplication you will see target folder, inside that you will get .jar file with name firstSpringBootApplication-0.0.1-SNAPSHOT.jar
Run the jar file of Application
Step 1: Go to this path C:\Users\1302143\Desktop\firstSpringBootApplication\target in the command prompt and type following command.
java -jar firstSpringBootApplication-0.0.1-SNAPSHOT.jar
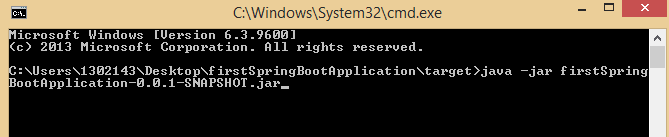
Step 2: Press Enter. Your application will be running on port 8080. You can check on localhost:8080 on your browser.
Generate the JSON Web Token through POSTMAN
Step 1: Open the POSTMAN and add the data in Authorization Section.
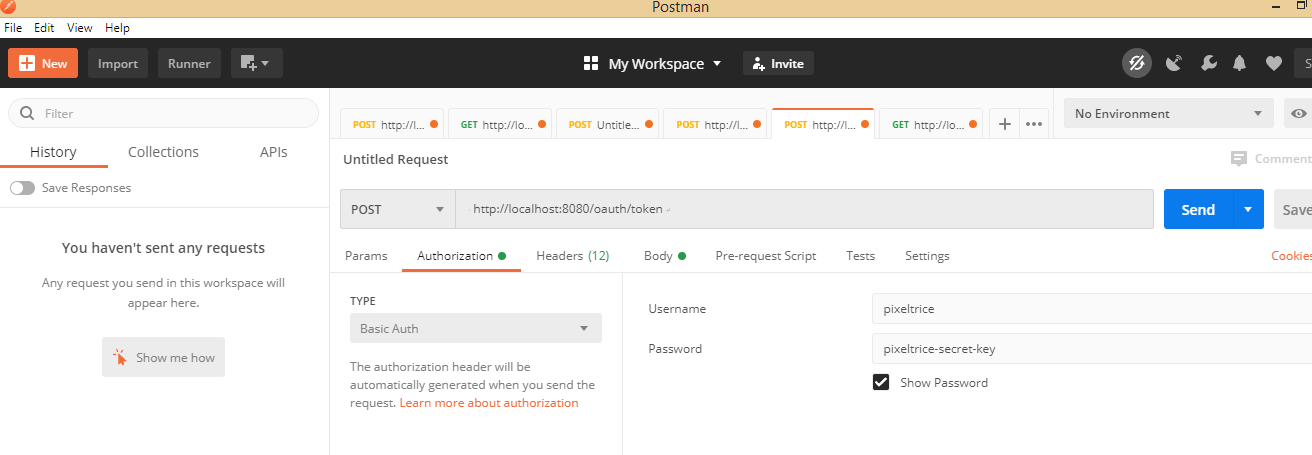
Inside this section select or fill following fields.
- Type: Choose Basic Auth from the dropdown.
- Username: Enter the clientId which you mentioned in ConfigOAuth.java class.
- Password: Add the client secret value which you already wrote inside ConfigOAuth.java class.
Step 2: Fill the data in Body Section of POSTMAN
Under this section select x-www-form-urlencoded. Now add the following key-value pair as shown in the figure.
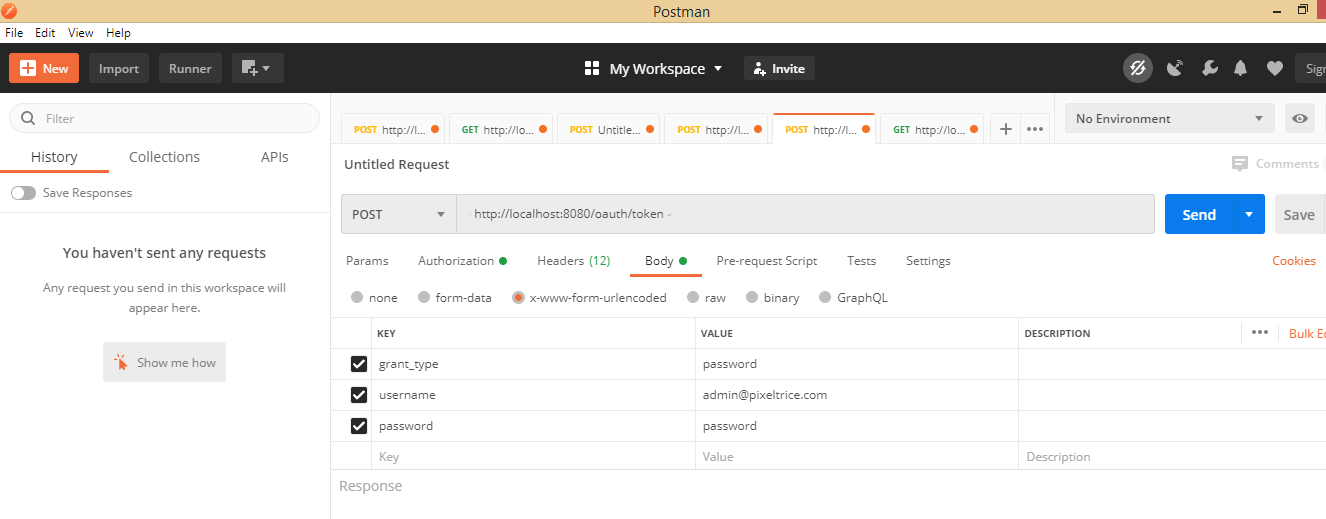
- grant_type: It should be a password keyword.
- username: username of the inserted value in the H2 database. Go to the data.sql file.
- password: It should be the password of users that you inserted in the database. You will get in data.sql, but their password is in Bcrypt format, the plain text of that format is the word password itself.
Step 3: Send the POST Request of API http://localhost:8080/oauth/token from POSTMAN, you will the token in response as shown in the figure.
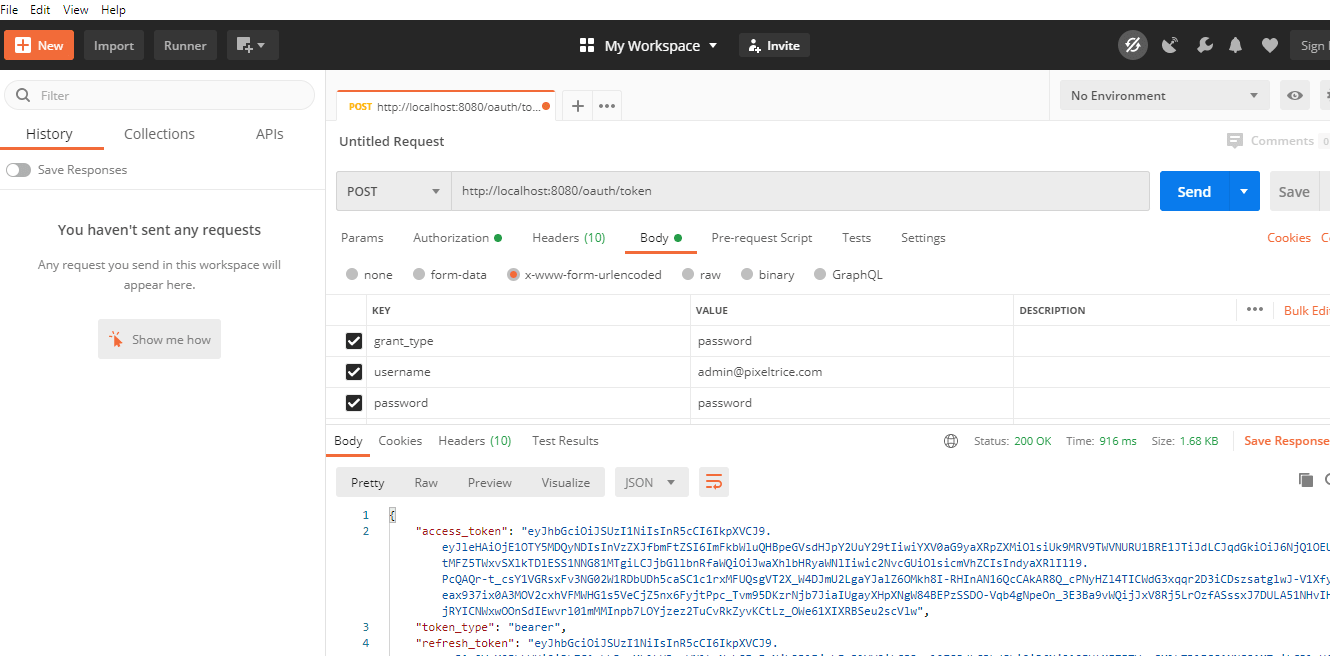
Step 4: Add the JSON Token inside the Header Section and also add the Content-Type as application/json as shown in the figure.
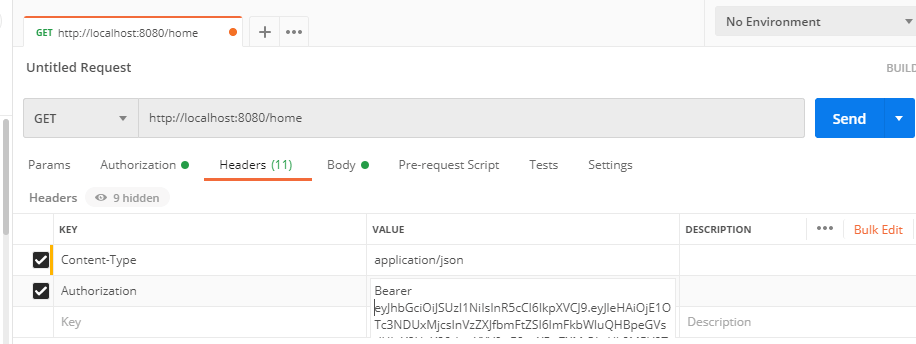
Note: Make sure that you should add token after Bearer in the Header section as shown in the above figure.
Step 5: Go to the Authorization tab in POSTMAN and change the Type from Basic Auth to Bearer Token as shown in the below figure.
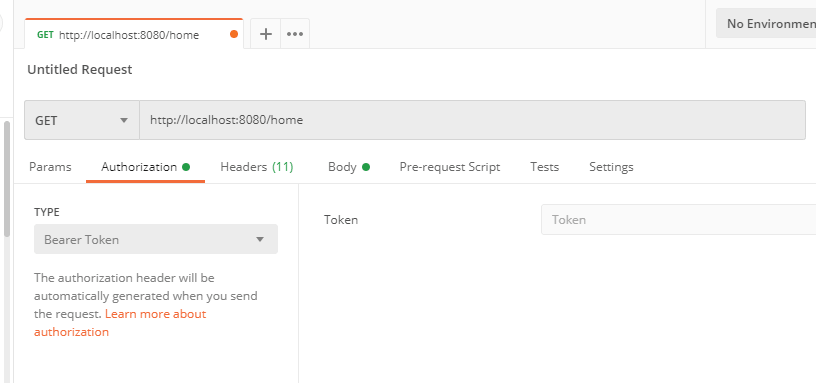
Step 6: Now Hit the endpoint of API http://localhost:8080/home URL to access the resources. You will now able to access the resource or API as shown in the figure.
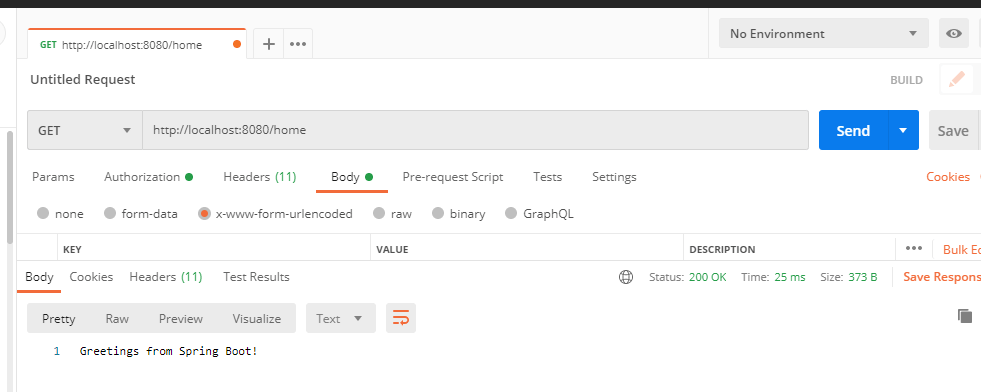
Source Code: Github link
Summary
In this article, we have learned to implement the Spring Boot Security using OAuth2 with JWT. If you have queries or doubts please feel free to ask in the comment section. I will love to answer your queries.
You can also checkout my other Spring Boot articles.
- How to send email using Spring Boot Application.
- Deploy the Spring Boot Application on External Tomcat Server
- How to develop a Spring Boot Web Application using Thymeleaf.
- How to add an Interceptor in a Spring Boot Application.
- Run Application from Spring Boot CLI.